In this laravel tutorial, we will learn about how to upload file in s3
You can follow these steps by step explain to you how to upload images in the s3. you can also upload files in s3 using laravel but in this article, we will upload the images in the s3 using laravel.
Step 1: Create S3 Bucket
You need to login your AWS account then search s3 from search box and follow bellow Setp:
1. Go to Amazon Web Service Console and Login.
2. Click on S3 from Service Lists
3. Click on “Create Bucket” button and you will found bellow forms. you can enter your bucket name there.
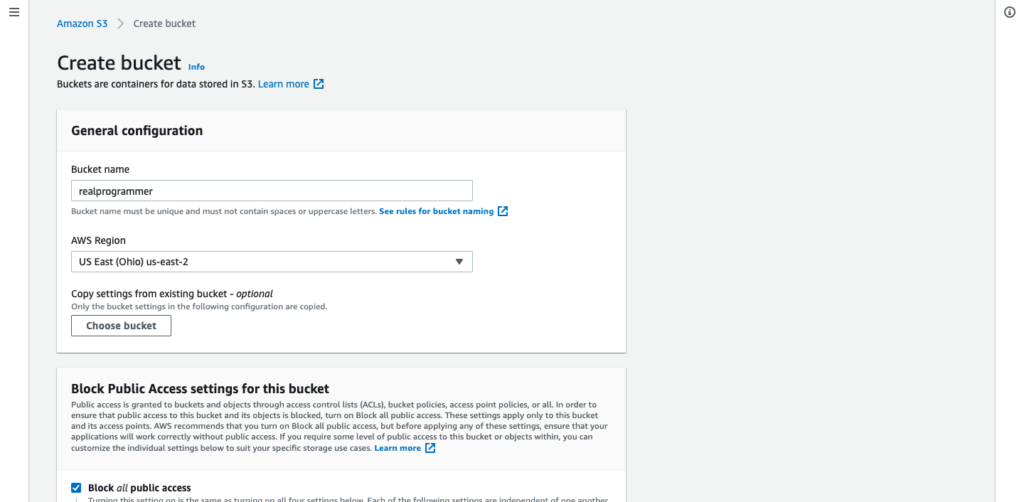
4. Create Create IAM User. Click here to create IAM User.
5. Click on “Create User” button as bellow show you.
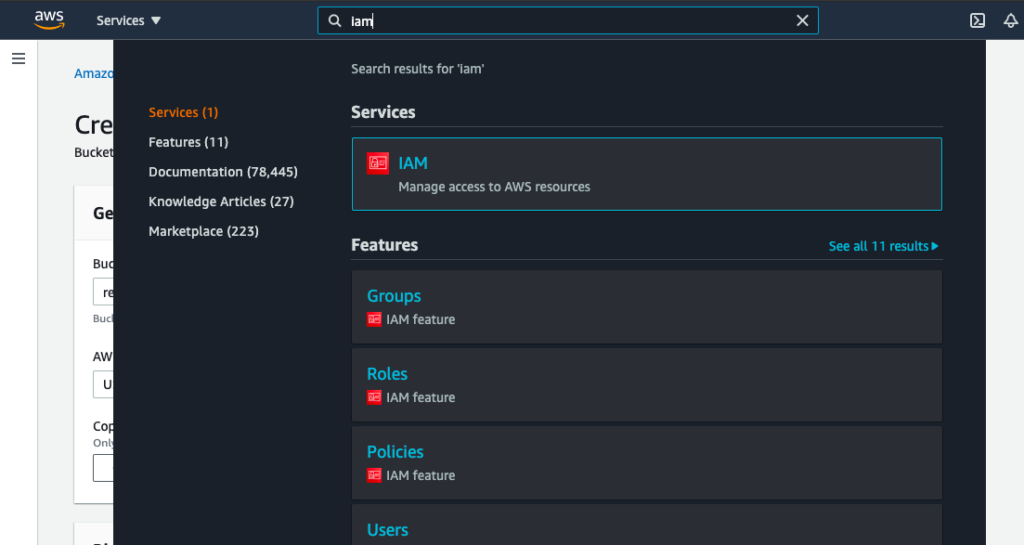
6. Next Add User Name and select “Programmatic access” from access type. then click on next.
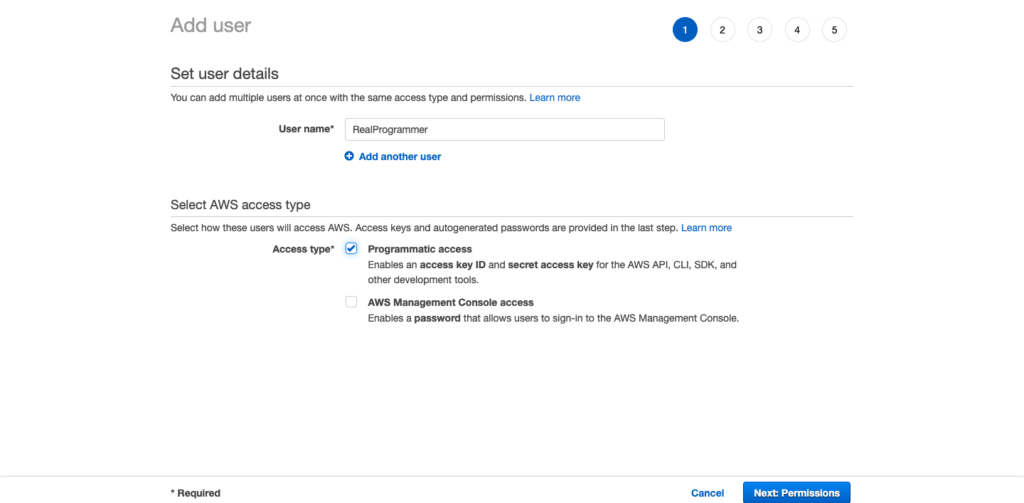
7. Next Select “Attach Existing Policy Directly” and choose “AmazonS3FullAccess” from permission link.
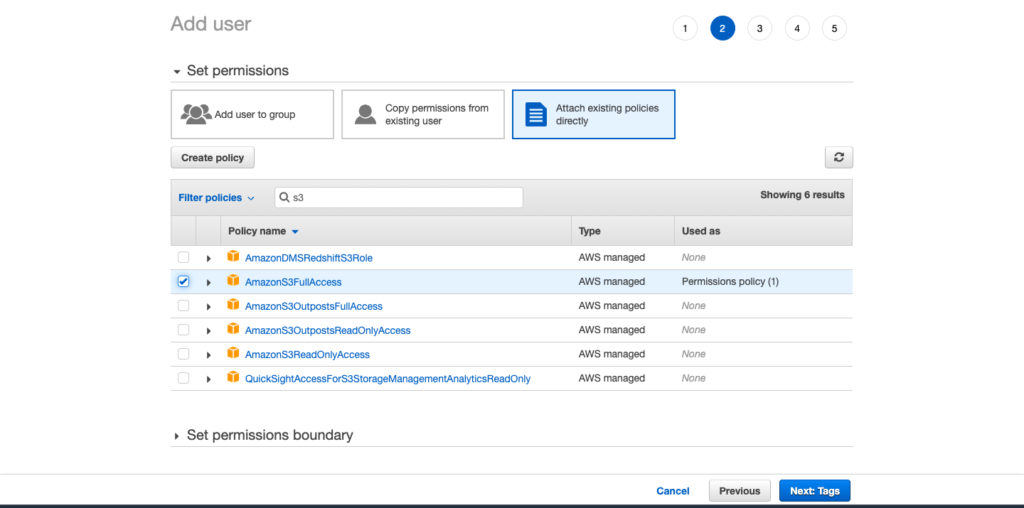
8. It’s optional so you can skip and click to next.
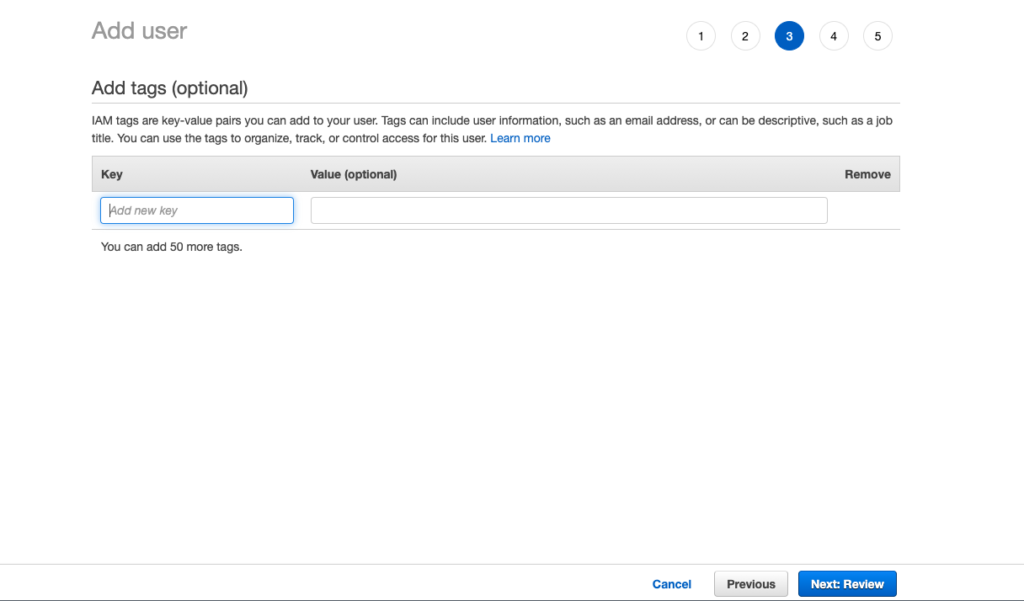
9. You can view user details and then click on “Create User” button.
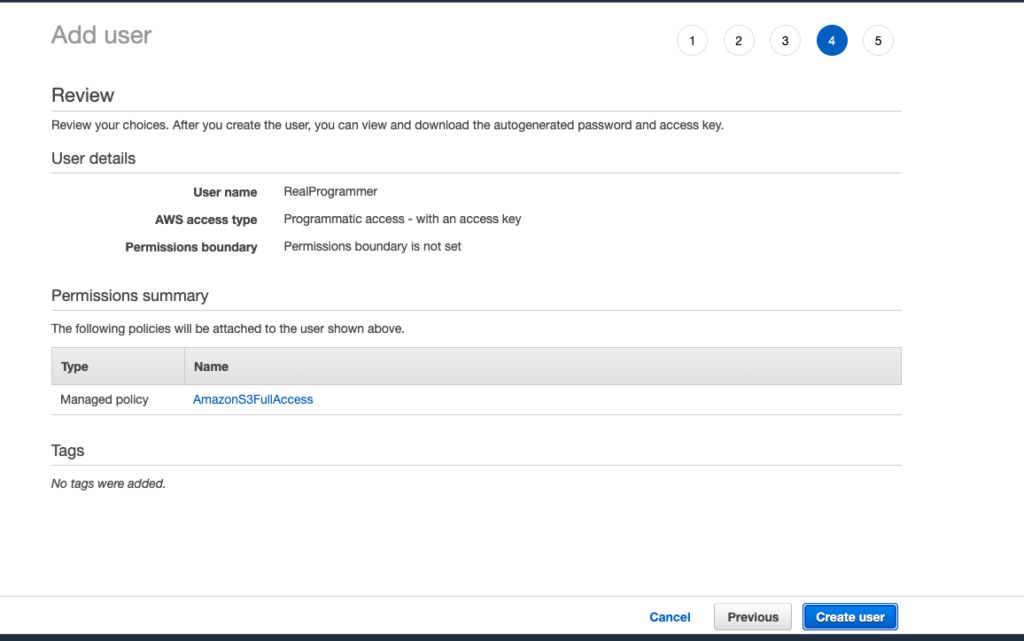
10. Now you will see created user in link. there is a “Access Key ID” and “Secret Access Key” that we need on .env files.
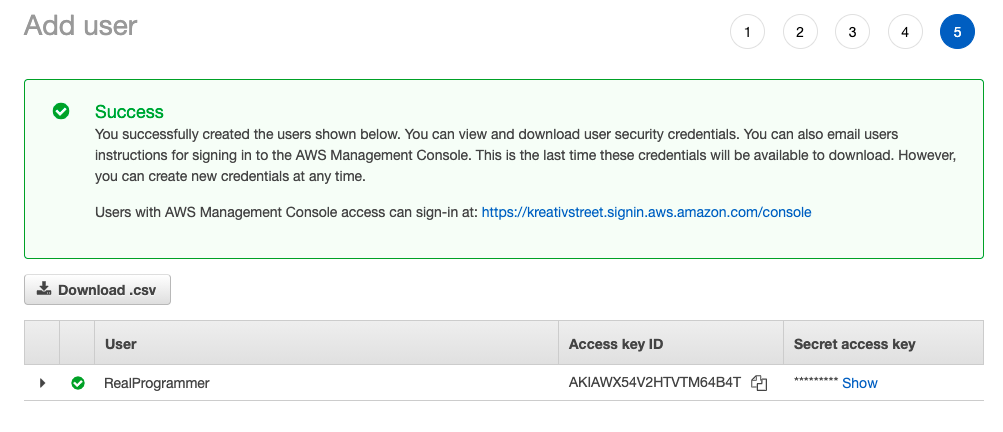
Step 2: Install Laravel 9
composer create-project --prefer-dist laravel/laravel laravelS3
cd laravelS3
Step 3: Install Amazon S3 Composer Package
composer require --with-all-dependencies league/flysystem-aws-s3-v3 "^1.0"
Step 4: Configure AWS S3 Credentials
AWS_ACCESS_KEY_ID=Your_AWS_ACCESS_KEY_ID
AWS_SECRET_ACCESS_KEY=Your_AWS_SECRET_ACCESS_KEY
AWS_DEFAULT_REGION=us-east-2
AWS_BUCKET=Your_AWS_BUCKET
AWS_USE_PATH_STYLE_ENDPOINT=false
Step 5: Create Routes
<?php
use Illuminate\Support\Facades\Route;
use App\Http\Controllers\FileUploadController;
Route::get('image-upload', [ FileUploadController::class, 'imageUpload' ])->name('image.upload');
Route::post('image-upload', [ FileUploadController::class, 'imageUploadPost' ])->name('image.upload.post');
Step 6: Create ImageUploadController
touch app/Http/Controllers/ImageUploadController.php
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
class FileUploadController extends Controller
{
/**
* Display a listing of the resource.
*
* @return \Illuminate\Http\Response
*/
public function imageUpload()
{
return view('imageUpload');
}
/**
* Display a listing of the resource.
*
* @return \Illuminate\Http\Response
*/
public function imageUploadPost(Request $request)
{
$request->validate([
'image' => 'required|image|mimes:jpeg,png,jpg,gif,svg|max:2048',
]);
$imageName = time().'.'.$request->image->extension();
$path = Storage::disk('s3')->put('images', $request->image);
$path = Storage::disk('s3')->url($path);
/* Store $imageName name in DATABASE from HERE */
return back()
->with('success','You have successfully upload image.')
->with('image', $path);
}
}
Step 7: Create Blade File
touch resources/views/imageUpload.blade.php
<!DOCTYPE html>
<html>
<head>
<title>Laravel 9 S3 File Upload Tutorial With Example </title>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0/css/bootstrap.min.css">
</head>
<body>
<div class="container">
<div class="jumbotron">
<h1>Laravel 0 S3 File Upload Tutorial With Example</h1>
</div>
<div class="panel panel-primary">
<div class="jumbotron">
<div class="panel-body">
@if ($message = Session::get('success'))
<div class="alert alert-success alert-block">
<button type="button" class="close" data-dismiss="alert">×</button>
<strong>{{ $message }}</strong>
</div>
<img src="{{ Session::get('image') }}" style="width:800px">
@endif
@if (count($errors) > 0)
<div class="alert alert-danger">
<strong>Whoops!</strong> There were some problems with your input.
<ul>
@foreach ($errors->all() as $error)
<li>{{ $error }}</li>
@endforeach
</ul>
</div>
@endif
<form action="{{ route('image.upload.post') }}" method="POST" enctype="multipart/form-data">
@csrf
<div class="row">
<div class="col-md-6">
<input type="file" name="image" class="form-control">
</div>
<div class="col-md-6">
<button type="submit" class="btn btn-success">Upload</button>
</div>
</div>
</form>
</div>
</div>
</div>
</body>
</html>
Step 8: Run Server
php artisan serve
http://127.0.0.1:8000/image-upload
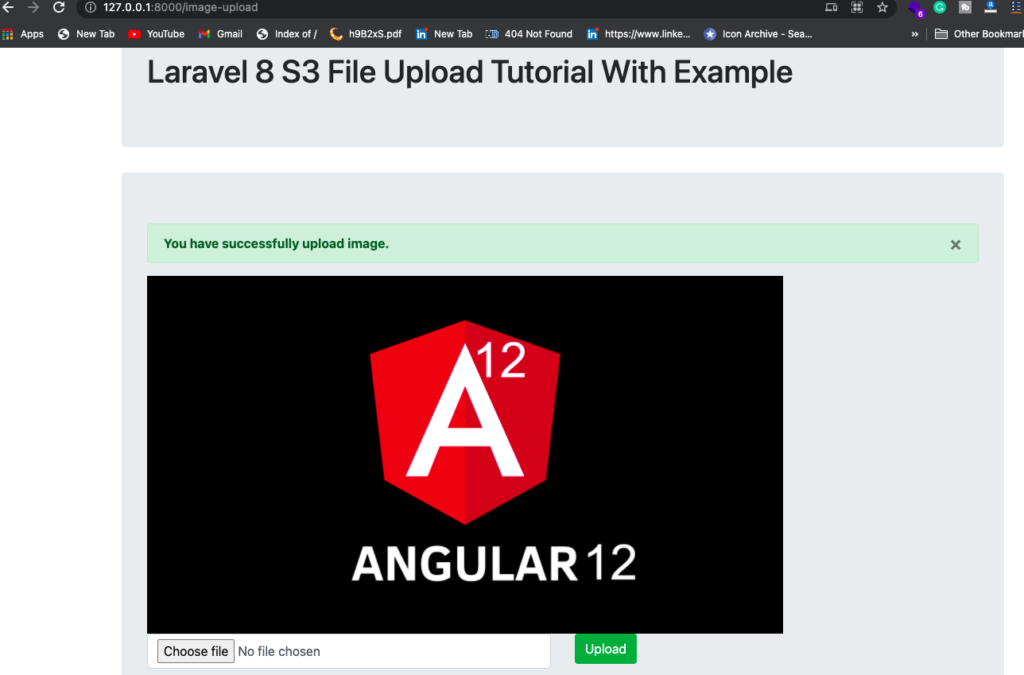
If you phasing problem to show image then you can change policy from s3 bucket.
{
"Version":"2012-10-17",
"Statement":[
{
"Sid":"PublicRead",
"Effect":"Allow",
"Principal": "*",
"Action":["s3:GetObject"],
"Resource":["arn:aws:s3:::realprogrammer/*"]
}
]
}
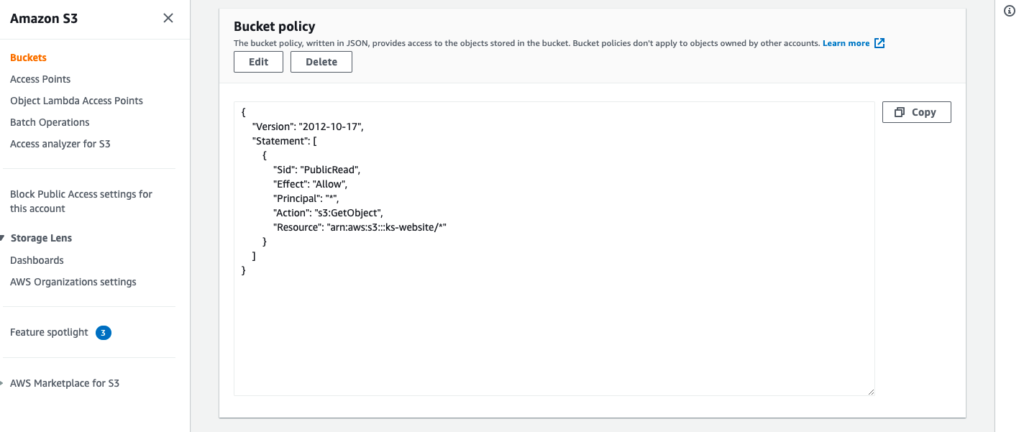
Git: https://github.com/siddharth018/LaravelS3FileUpload